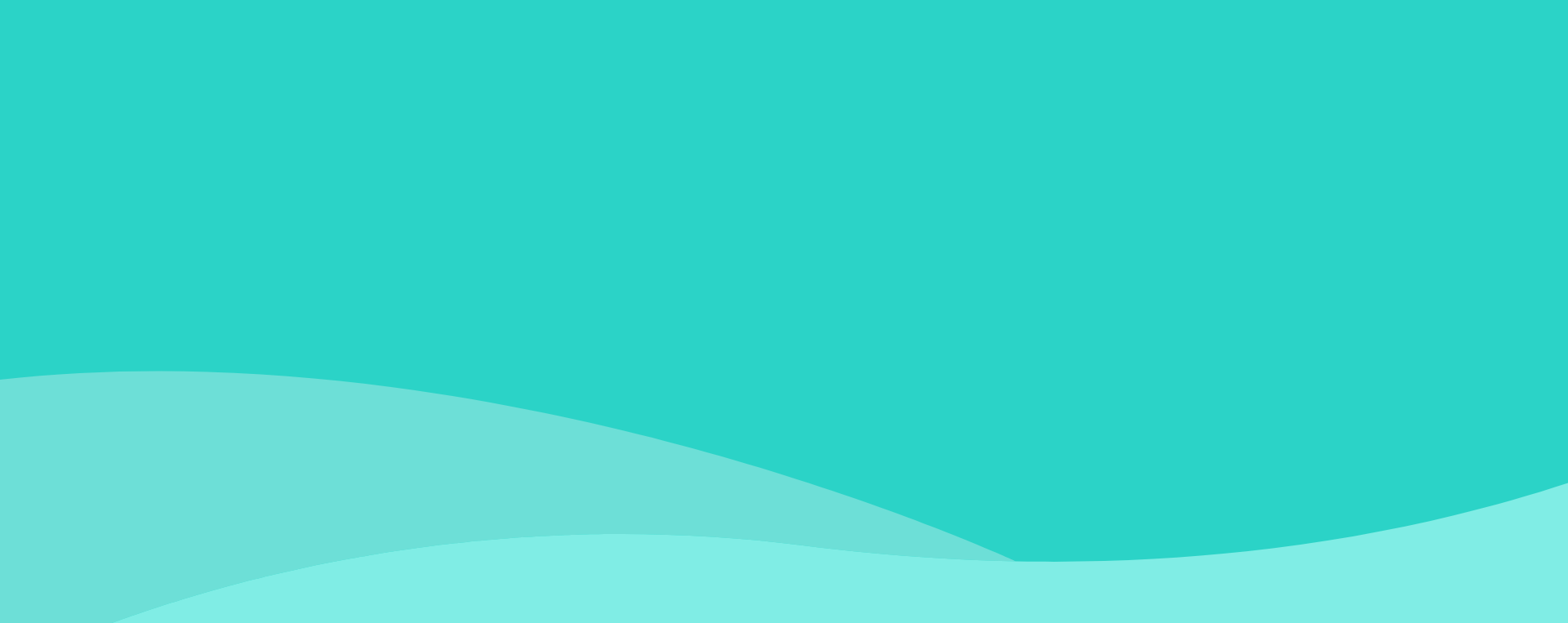
HaloITSM Guides
Documentation to assist with the setup and configuration of the HaloITSM platform
Halo Chat Widget Properties and Examples (v2.172+)
Quickstart
You can embed chat on your website by adding the following to the end of your html body. You can retrieve your chat config id and key from Config > Chat > Chat Profiles, in the Halo Agent Application.
<div id="halo-chat"></div>
<script>
var haloChatConfig = {
chatServiceUrl: "https://yourHaloUrl.com/api",
configId: "your-chat-config-id",
configKey: "your-chat-config-key"
}
</script>
<script src="https://yourHaloUrl.com/chat/index.js"></script>
Props
All the basic props are listed here.
Property | Type | Mandatory | Description |
---|---|---|---|
chatServiceUrl | string | true | Your Halo API url. |
tenantId | string | false | Hosted Halo only. Your unique tenant id found in Config > Integrations > Halo API. |
configId | string | true | The Id of the chat profile record you want to use. Found in Config > Chat > Chat Profiles. |
configKey | string | true | The Key of the chat profile record you want to use. Found in Config > Chat > Chat Profiles. |
colour | string | false | The primary colour of the chat bubble and window. Accepts a HEX colour. E.g #E83C4A. |
theme | string | false | Accepts "light" or "dark". Default is light. |
persistChatToken | bool | false | When using chat anonymously, a chat access token will be created when you create a chat. This property determines if the token (and hence chat session) will persist between refreshes by storing the token as a cookie. Defaults to true. |
chatMode | string | false | Agent chat only. For user chat this is determined by the global setting in Halo. Accepts "heartbeat" or "websocket". Default is "websocket". |
height | int | false | The height of the chat window |
width | int | false | The width of the chat window |
sound | string | false | Set to the url of a sound file to override the default new message sound. |
icon | string | false | Set to the url of an image to change the default icon on the chat bubble. |
iconSize | int | false | The size of the icon as a width/height percentage of the bubble. Default is 50. |
frameInheritHead | bool | false | If true, the chat window frame will inherit the html head from the document that renders the frame. |
includeFont | bool | false | If false, the google font Poppins will not be loaded. Default is true. |
language | object | false | Used to override language used in Halo Chat. See Language below. |
debug | bool | false | When set to true, additional logging will show in the console. |
keepChatWindowOpen | bool | false | When set to true the chat window will remain open between page refreshes if it was already open. This uses session storage to preserve it's state. |
position | string | false | This determines the default position of the bubble and conversation. Possible values are "top-left", "top-right", "bottom-left", "bottom-right" |
bubbleContainerStyles | style object | false | Use this to pass custom styles to the bubble container. E.g { bottom: "100px", right: "100px" } will reposition it. |
bubbleStyles | style object | false | Use this to pass custom styles to the bubble. |
windowStyles | style object | false | Use this to pass custom styles to the message window. E.g { bottom: "100px", right: "100px" } will reposition it. |
showEndChatOptionOnBubble | bool | false | When set to true this will provide an option to end chat from the bubble. This shows on hover and when clicked on will prompt the user for confirmation before ending the chat. |
forceFullScreen | bool | false | When set to true make the chat window always use full screen and remove the option to toggle full screen mode. |
areYouStillThereReopenChat | bool | false | When the user is prompted to confirm they are still there, this will reopen the chat window automatically if it's minimized. |
hideNewChatBubble | bool | false | When set to true the chat bubble will not show if there is not an open conversation. This should be used with the events API. |
hideExistingChatBubble | bool | false | When set to true the chat bubble will not show if there is an open conversation. This should be used with the events API. |
autoInitialize | bool | false | When set to false chat will not start or make any Halo API calls until it is initialized by an event. This should be used with the events API. |
draggableBubble | bool | false | When set to true the chat bubble is draggable and can be repositioned. This should be used with the events API. |
retainPreviousChat | bool | false | When a new chat is launched with an id_token, if this is true and the user has a old chat which is still open, this chat will be resumed. This should be used with the events API. |
hideBubbleInactivitySeconds | int | false | When set to a number > 0, the bubble will become hidden if it's not clicked on for the number of seconds specified. In order to show it again the events API must be used to re-open the chat window. |
enableInboundEvents | bool | false | When set to true Halo chat will can receive javascript events to control it. This should be used with the events API. |
enableOutboundEvents | bool | false | When set to true Halo chat will send javascript events when particular things happen (chat initialized, window opened, window closed). This should be used with the events API. |
enableFunctions | bool | false | When set to true Halo chat will allow specific functions to be executable from outside of Halo chat. E.g window.haloChat_functions_getCurrentChatDetails(). |
hideHeader | bool | false | When set to true the Halo Chat header will be hidden. You will need to implement your own functions to call the events to open/close the chat menu (haloChat_inboundEvent_toggleMenu), and for the user to end the chat (haloChat_inboundEvent_endChat). |
Language
Below you'll find the default language object. This can be customised by parsing the language prop.
const language = {
newChatName: 'Start a new Chat',
liveChatName: 'Live Chat',
ticket: 'Ticket',
ended: 'Ended',
unreadCount: '@@@ unread message(s)',
remove: 'Remove',
dangerousMessage:
'Content has been blocked due to being potentially dangerous',
loadOld: 'Load older messages',
chatDetails: 'Chat Details',
save: 'Save',
cancel: 'Cancel',
close: 'Close',
namePlaceholder: 'Enter a name for this Chat',
copyTranscript: 'Copy Transcript',
endChat: 'End this Chat',
restartChat: 'Restart Chat',
participants: 'Participants',
add: 'Add',
sendCanned: 'Send a Canned Message',
messagePlaceholder: 'Type your message and press Enter to send',
changeIcon: 'Change Icon',
send: 'Send',
newChatRequest: 'New Chat Request',
isTyping: '@@@ is typing',
waitingForAgent: 'Waiting for an Agent to join'
}
Events API
The Events API allows you to control the chat widget using Javascript events.
For example you can hide the new chat bubble and open a new conversation on click of a button on your website.
Inbound Events
Enable enableInboundEvents
to use these.
Event Name | Arguments | Description |
---|---|---|
haloChat_inboundEvent_initialize | null | Send this event to initialize chat when autoInitialize is false. |
haloChat_inboundEvent_openChatWindow | { id_token: "idTokenValue", retainPreviousChat: bool } | If there is not currently a conversation in progress, this function will begin a new chat with the identity token specified, and open the conversation window. If there is already a conversation in progress, it will open the conversation window only and not use the Identity Token. The id_token is an optional argument to allow the conversation to be authenticated as a user. retainPreviousChat can be parsing to override the value of the retainPreviousChat prop. |
haloChat_inboundEvent_closeChatWindow | null | Send this event to close an open chat window. |
haloChat_inboundEvent_loginStateChanged | null | Send this event to clear the current chat session and connection. |
haloChat_inboundEvent_toggleFullScreen | bool | Send this event with or to toggle full screen of an open chat window. |
haloChat_inboundEvent_resize | { width: w, height: h } | Send this event to resize the chat window, specifying a new width and height in pixels. |
haloChat_inboundEvent_reposition | "position" | Override the value for the "position" property using an event. E.g "bottom-left". |
haloChat_inboundEvent_repositionBubble | { x: x, y: y } | Reposition the bubble on the screen. To be used with drag and drop (draggableBubble property). |
haloChat_inboundEvent_upgradeAnonChat | { id_token: "idTokenValue" } | Send this event if there was previously an anonymous chat session, and the user has since authenticated. Supply an id_token for the new user and the chat will be reassigned to the matching User account in Halo and now be classed as Authenticated chat. The result can be returned using the haloChat_outboundEvent_upgradeAnonChat event. |
haloChat_inboundEvent_toggleMenu | null | Send this event to open/close the chat menu on a toggle. |
haloChat_inboundEvent_endChat | null | Send this event open the end chat confirm screen for the user to end a chat. |
Outbound Events
Enable enableOutboundEvents
to use these.
Event Name | Arguments | Description |
---|---|---|
haloChat_outboundEvent_initialized | Sent when chat has finished initializing and is ready to use. chatAvailable will be true if it's ready to use, false if it's not available due to configuration such as chat workday availability. | |
haloChat_outboundEvent_chatOpened | obj | Sent when the chat window is opened. The chat header object is sent. |
haloChat_outboundEvent_chatClosed | null | Sent when the chat window is closed. |
haloChat_outboundEvent_newMessage | obj | Sent when a new message is received. This sends the message object. |
haloChat_outboundEvent_upgradeAnonChat | { success: bool, error: string } | Sent when attempting to upgrade an anonymous chat to authenticated using the haloChat_inboundEvent_upgradeAnonChat inbound event. If it was successful, success will be returned as true. If unsuccessful the reason will be returned in the error property. |
haloChat_outboundEvent_newChatStarting | null | Sent when the user begins a new chat session (before the chat has been saved). |
haloChat_outboundEvent_newChatStarted | obj | Sent when the user begins a new chat session (after the chat has been saved). Sends the chat header object. |
haloChat_outboundEvent_existingChatResumed | obj | Sent when the user resumes an existing chat session. Sends the chat header object. |
haloChat_outboundEvent_messageSent | obj | Sent when the user sends a message. This sends a chat message object. |
haloChat_outboundEvent_chatEnded | obj | Sent when the chat has ended. This sends the chat header object. |
haloChat_outboundEvent_awaitingAgent | obj | Sent when an chat session starts waiting for an agent to join. This sends the chat header object. |
haloChat_outboundEvent_agentJoined | obj | Sent when an agent joins a chat session. This sends the chat header object. |
haloChat_outboundEvent_userTyping | null | Sent when the user starts typing. |
haloChat_outboundEvent_userStoppedTyping | null | Sent when the user stops typing and clears their message. |
haloChat_outboundEvent_imStillHereConfirm | null | Sent when the user is prompted to confirm they still require assistance. |
haloChat_outboundEvent_imStillHereConfirmed | null | Sent when the user confirms they still require assistance. |
Functions
These are functions you can call to retrieve data about the current state of the Chat Widget.
Enable enableFunctions
to use these.
Function Name | Arguments | Output | Description |
---|---|---|---|
haloChat_functions_getCurrentChatDetails | n/a | [obj] | Call this function to retrieve the current chat data from the Chat Widget. This will return an array of conversations, which will be empty if there are no open conversations, or contain a chat header object if there is an open conversation. This will contain attributes such as the chat id (id), unread message count (unread_count), and an array of messages (messages). |
Example
The following example page demonstrates usage of the Events API.
It initializes when required, and has the new conversation bubble hidden using hideNewChatBubble
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta
name="viewport"
content="width=device-width, initial-scale=1, shrink-to-fit=no"
/>
<meta name="theme-color" content="#000000" />
<title>Halo Chat Widget Events API Example</title>
<style>
.hint {
font-size: 14px;
}
.control-box {
border: 1px solid black;
padding: 0 20px 20px;
width: calc(100% - 40px);
margin-bottom: 20px;
}
</style>
</head>
<body>
<noscript>
You need to enable JavaScript to run this app.
</noscript>
<div id="root">
<div class="control-box">
<h2>Intializing Chat</h2>
<ul class="hint">
<li>When 'autoInitialize' = false, Chat will not make any calls to the Halo API until the intialize event is sent.</li>
<li>This function sends the "haloChat_inboundEvent_initialize" event.</li>
<li>When chat is ready it will respond with event "haloChat_outboundEvent_initialized" and event.detail.chatAvailable will either be true or false depending on if the Chat Profile is available at the current time.</li>
<li>Chat will still auto-initialize if there is an ongoing conversation when the page refreshes.</li>
</ul>
<button onclick="initChat()">
Initialize Chat
</button>
<div style="margin-top: 10px;">
<span id="connectionStatusContainer" style="display: inline-flex; align-items: center; margin-left: 10px;">
<div id="statusIndicator" style="width: 10px; height: 10px; border-radius: 50%; background-color: grey; margin-right: 5px;"></div>
<span id="connectionStatus">Not connected</span>
</span>
</div>
</div>
<div class="control-box">
<h2>Identity Token Management and Opening Chat</h2>
<ul class="hint">
<li>The Open Chat button sends the "haloChat_inboundEvent_openChatWindow" event to chat, along with the Identity Token JWT entered below. If there is not currently a conversation in progress, this function will begin a new chat with the identity token specified, and open the conversation window. If there is already a conversation in progress, it will open the conversation window only and not use the Identity Token.</li>
<li>The Identity token is optional - if not specified the chat will behave as if you are not logged in. If specified it will either create a user or match to an existing user in Halo, and act as if you are authenticated as that user.</li>
<li>When a new conversation is created with an Identity token a Chat session cookie will be issued and that is used to retain access to the conversation.</li>
<li>The "Upgrade Anon Chat to Authenticated Chat" button sends the "haloChat_inboundEvent_upgradeAnonChat" event to chat. This expects an Identity token. If there is an open Anonymous chat, this will upgrade the chat to an Authenticated Chat using the identity token. This page listens to the haloChat_outboundEvent_upgradeAnonChat event to report the result.</li>
<li>The "Login Changed / Logout / Clear Session" button sends the "haloChat_inboundEvent_loginStateChanged" event to chat. This effectively ends the current session, removing the conversation and Chat session cookie, allowing you to then open a new conversation with a different identity token. This function should be called when the authenticated user changes or logs out.</li>
</ul>
<div style="margin-top: 20px;">
<label for="id_token">Identity Token JWT:</label><br>
<span style="font-size: 11px">This will get passed to the New Chat / Open Chat Window function (when there isn't an open conversation), and the Upgrade Anon Chat to Authenticated Chat function.</span><br>
<textarea id="id_token" rows="4" cols="50" autocomplete="off" placeholder="Paste JWT here..."></textarea>
</div>
<div style="margin-top: 30px;">
<button onclick="openChat()" disabled>
New Chat / Open Chat Window
</button>
<button onclick="upgradeAnonChat()" disabled>
Upgrade Anon Chat to Authenticated Chat
</button>
<button onclick="loginStateChanged()" disabled>
Login Changed / Logout / Clear Session
</button>
</div>
</div>
<div class="control-box">
<h2>Chat Controls</h2>
<ul class="hint">
<li>These are additional functions that allow you to control the chat window using Javascript events</li>
<li>You can also drag the Chat bubble around this page. This uses the "haloChat_inboundEvent_repositionBubble" to update it's position.</li>
</ul>
<div style="margin-top: 30px;">
<button onclick="openChat()" disabled>
Open Chat Window
</button>
<button onclick="closeChat()" disabled>
Close Chat Window
</button>
</div>
<div style="margin-top: 30px;">
<button onclick="fullScreenOn()" disabled>
Full-screen on
</button>
<button onclick="fullScreenOff()" disabled>
Full-screen off
</button>
</div>
<div style="margin-top: 30px;">
<button onclick="toggleMenu()" disabled>
Toggle Menu
</button>
</div>
<div style="margin-top: 30px;">
<button onclick="endChat()" disabled>
End Chat
</button>
</div>
<div style="margin-top: 30px;">
<label for="width">Window Width:</label><br>
<input id="width" autocomplete="off" placeholder="400" style="width: 100px"></input><br>
<label for="height">Window Height:</label><br>
<input id="height" autocomplete="off" placeholder="700" style="width: 100px"></input><br>
<button onclick="resize()" disabled>
Resize
</button>
<br><br>
<button onclick="reposition('top-left')" disabled>
Top-Left
</button>
<button onclick="reposition('top-right')" disabled>
Top-Right
</button>
<br>
<button onclick="reposition('bottom-left')" disabled>
Bottom-Left
</button>
<button onclick="reposition('bottom-right')" disabled>
Bottom-Right
</button>
</div>
</div>
<div class="control-box">
<h2>Getting Chat Data</h2>
<ul class="hint">
<li>This demonstrates how to get the information regarding the current state of Halo Chat.</li>
<li>"Get Chat Data" calls a function in Halo Chat to retrieve information on the current open chat, such as unread count, and messages.</li>
<li>When new messages are received Halo Chat uses the haloChat_outboundEvent_newMessage outbound event to send this page information about the new message.</li>
<li>Data from additional outbound events will show in the Javascript console.</li>
</ul>
<div style="margin-top: 30px;">
<button onclick="getChatData()" disabled>
Get Chat Data
</button>
<br>
<textarea id="chat_data" disabled rows="10" cols="100" autocomplete="off" placeholder="Chat Data will show here..."></textarea>
</div>
<div style="margin-top: 30px;">
New message data will show here
<br>
<textarea id="new_messages" disabled rows="10" cols="100" autocomplete="off" placeholder="New messages will show here..."></textarea>
</div>
</div>
<script>
window.addEventListener("haloChat_outboundEvent_initialized", function(event) {
console.log("Chat initialized event received:", event);
const buttons = document.querySelectorAll("#root button");
if (event.detail?.chatAvailable) {
document.getElementById("connectionStatus").textContent = "Started";
document.getElementById("statusIndicator").style.backgroundColor = "green";
buttons.forEach(button => button.disabled = false);
} else {
document.getElementById("connectionStatus").textContent = "Chat not available";
document.getElementById("statusIndicator").style.backgroundColor = "red";
buttons.forEach(button => button.disabled = true);
}
});
function initChat() {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_initialize"));
}
function openChat() {
const idToken = document.getElementById("id_token").value;
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_openChatWindow", { detail: { id_token: idToken, retainPreviousChat: true } }));
document.getElementById("id_token").value = "";
}
function upgradeAnonChat() {
const idToken = document.getElementById("id_token").value;
if (!idToken) {
window.alert("id_token is required.")
} else {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_upgradeAnonChat", { detail: { id_token: idToken } }));
document.getElementById("id_token").value = "";
}
}
function loginStateChanged() {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_loginStateChanged"));
}
function closeChat() {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_closeChatWindow"));
}
function fullScreenOn() {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_toggleFullScreen", { detail: true }));
}
function fullScreenOff() {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_toggleFullScreen", { detail: false }));
}
function resize() {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_resize", { detail: { width: document.getElementById("width").value, height: document.getElementById("height").value}} ));
}
function reposition(position) {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_reposition", { detail: position } ));
}
function toggleMenu() {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_toggleMenu"));
}
function endChat() {
window.dispatchEvent(new CustomEvent("haloChat_inboundEvent_endChat"));
}
function getChatData() {
if (typeof(window.haloChat_functions_getCurrentChatDetails) === 'function') {
var data = haloChat_functions_getCurrentChatDetails();
document.getElementById("chat_data").value = JSON.stringify(data, null, 2);
} else {
window.alert("Function not available");
}
}
window.addEventListener("haloChat_outboundEvent_newMessage", function(event) {
console.log("New message event received:", event?.detail);
var el = document.getElementById("new_messages");
if (el.value) {
el.value += '\n\n'
}
el.value += JSON.stringify(event.detail, null, null);
el.scrollTop = el.scrollHeight;
});
window.addEventListener("haloChat_outboundEvent_upgradeAnonChat", function(event) {
console.log("upgrade Anon Chat result:", event);
var result = event.detail.success;
var error = event.detail.error;
window.alert(`upgrade result=${result}, error=${error}`);
});
const dropContainer = document.getElementById('root');
dropContainer.addEventListener('dragover', (event) => {
event.preventDefault();
});
dropContainer.addEventListener('drop', (event) => {
event.preventDefault();
const rect = dropContainer.getBoundingClientRect();
const x = event.clientX - rect.left;
const y = event.clientY - rect.top;
window.dispatchEvent(new CustomEvent('haloChat_inboundEvent_repositionBubble', { detail: { x: x, y: y } }));
});
window.addEventListener("haloChat_outboundEvent_newChatStarting", function(event) {
eventReceived("haloChat_outboundEvent_newChatStarting", event);
});
window.addEventListener("haloChat_outboundEvent_newChatStarted", function(event) {
eventReceived("haloChat_outboundEvent_newChatStarted", event);
});
window.addEventListener("haloChat_outboundEvent_existingChatResumed", function(event) {
eventReceived("haloChat_outboundEvent_existingChatResumed", event);
});
window.addEventListener("haloChat_outboundEvent_messageSent", function(event) {
eventReceived("haloChat_outboundEvent_messageSent", event);
});
window.addEventListener("haloChat_outboundEvent_chatEnded", function(event) {
eventReceived("haloChat_outboundEvent_chatEnded", event);
});
window.addEventListener("haloChat_outboundEvent_awaitingAgent", function(event) {
eventReceived("haloChat_outboundEvent_awaitingAgent", event);
});
window.addEventListener("haloChat_outboundEvent_agentJoined", function(event) {
eventReceived("haloChat_outboundEvent_agentJoined", event);
});
window.addEventListener("haloChat_outboundEvent_userTyping", function(event) {
eventReceived("haloChat_outboundEvent_userTyping", event);
});
window.addEventListener("haloChat_outboundEvent_userStoppedTyping", function(event) {
eventReceived("haloChat_outboundEvent_userStoppedTyping", event);
});
function eventReceived(eventName, eventData) {
console.log(`${eventName} event received:`, eventData?.detail);
}
</script>
</div>
<div id="halo-chat"></div>
<script>
var haloChatConfig = {
chatServiceUrl: "https://yourHaloUrl.com/api",
configId: "your-chat-config-id",
configKey: "your-chat-config-key",
debug: false,
autoInitialize: false,
enableInboundEvents: true,
enableOutboundEvents: true,
enableFunctions: true,
hideNewChatBubble: true,
hideExistingChatBubble: false,
keepChatWindowOpen:true,
retainPreviousChat: true,
draggableBubble: true,
showEndChatOptionOnBubble: true,
hideBubbleInactivitySeconds: 300,
position: 'bottom-right',
hideHeader: false,
forceFullScreen: false,
bubbleContainerStyles: {
bottom: "20px",
right: "20px"
},
windowStyles: {
bottom: "20px",
right: "20px"
},
}
</script>
<script src="https://yourHaloUrl.com/chat/index.js"></script>
</body>
</html>
Popular Guides
- Asset Import - CSV/XLS/Spreadsheet Method
- Call Management in Halo
- Creating a New Application for API Connections
- Creating Agents and Editing Agent Details
- Departments and Teams
- Halo Integrator
- Importing Data
- Multiple New Portals with different branding for one customer [Hosted]
- NHServer Deprecation User Guide
- Organisation Basics
- Organising Teams of Agents
- Step-by-Step Configuration Walk Through
- Suppliers